Comments Section
The Idea
Over the last week I have been on a course namely 'Website fundimentals' where we are learning the basics of HTML, CSS and JS.
As I have previously learned most of the content for the first four days I decided to give myself a challange to complete over these days.
In the past I had an idea to add a comments section to the articles on my website (like this one).
My Plan
I created a list of features which would be required to complete the project:
- Build login / sign up forms
- Verify user emails
- Create a 'signed in strip' so users know when they are logged in
- Design the look of a comment
- API to handle comment storage
The API
For the API to be fully functional I needed to create the following endpoints:
- Create comment stream
- GET all comments from stream
- POST a comment to a stream
- PUT - Edit a comment
- DELETE a comment
- Vote - up and down
Integrating user login
I had already created a user authentication API.
The app uses JWT (JSON web token) authentication. This works by creating a random token when a user successfully logs in. The token is saved n their browser and will allow them access for an amount of time until the token expires and they must log in again.
I designed the pages and connected them to the API and it worked straight away, that would've been the most difficult part if I hadn't created the authentication API previously. The signed in state is below:

I then added a Google Recaptcha checkbox to prevent spam on my site, I also added states for when users are signed in but their email is not verified:

After logging in a user will be brought back to the page they started the login/sign up process from.
The pages for the login/sign up pages are below:
Comments Section Design
I first had to think about what data a comment would need. This would be the data model used by MongoDB and Typescript. The data model I settled with is below:
- ID
- Text
- Full Name
- Time
- upvotes
- downvotes
- hasBeenEdited
I then created the markup for a comment and styled it using SCSS:

When a user is not signed in they will see this state:
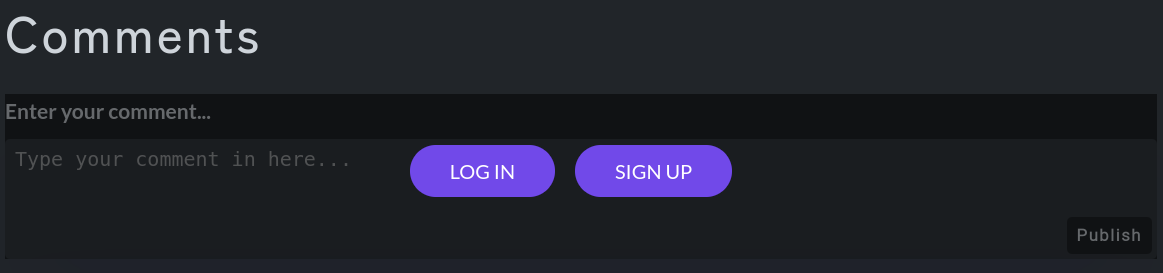
When a user is signed in, but their email is not verified they see this state:
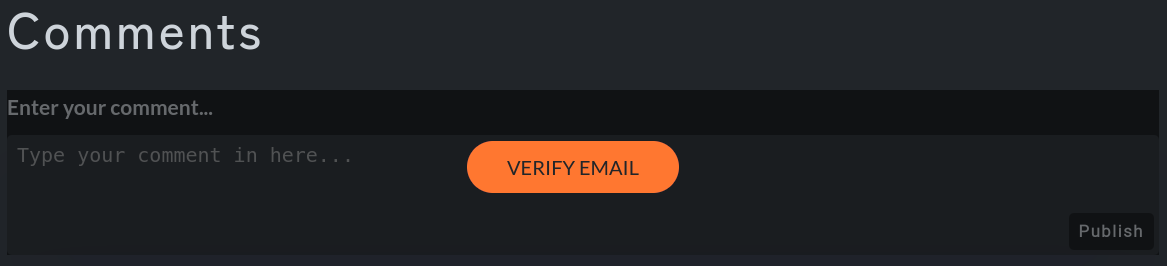
If a user is signed in and their email is verified they see the comment input box:
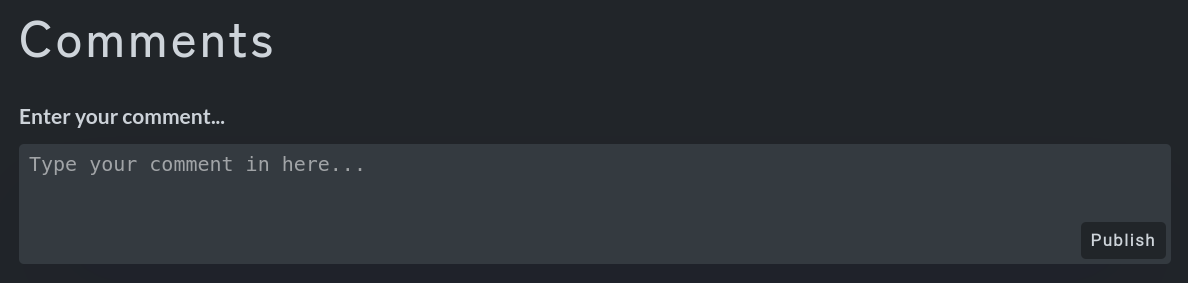
All of the designs are responsive so they work on all devices.
The Comment API
For the API, I wrote the code using Express (A NodeJS library) and Mongoose (A MongoDB library).
I added a folder into my API server repository and created all of the required endpoints, I used Postman to send requests to the API while developing it.
All of the endpoints use the JWT token to ensure that only verified users can comment.
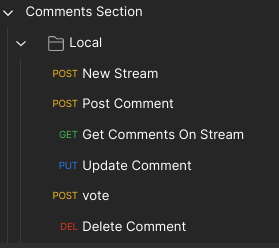
The API is running on a Linode cloud based server. I have configured the server to use HTTPS and with the E-Mail ports allowed so I can send email verification codes to users via SendGrid.
The database is running on a MongoDB Atlas Cloud which is a free service up to a certain amount of traffic or data stored.
The Frontend Logic
For the rendering of comments along with the user interface interactions, I chose to go with TypeScript (TS). I find TS to be less error prone and easier to code with due to the in editor error checking.
I wrote logic to call the GET all on stream endpoint, then it will use the data and render it to the page using the JS InsertAdjacentHTML method.
Each rendered comment has an ID added to the DataSet property, this is used when editing or deleting a comment.
Users can click the edit button on their comment if they are logged in which changes the look of the comment:
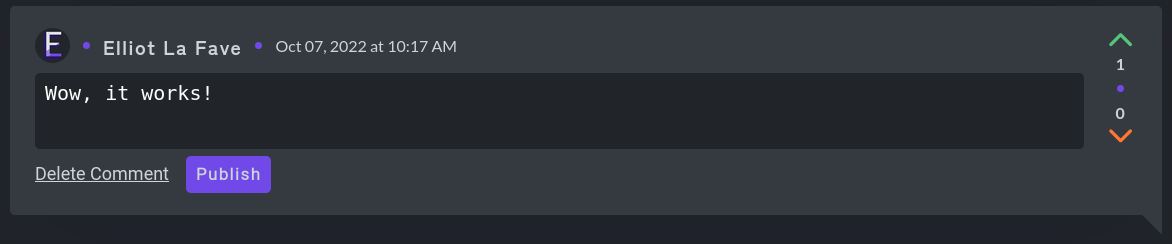
When editing users can use the DELETE endpoint and the PUT (update) endpoint.
No comments yet.